Writing 1st program of C#
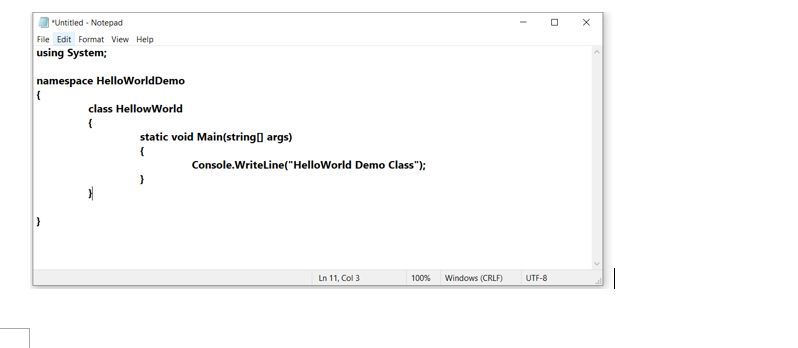
Rules to be followed. You need to produce high quality C# Code. Here are some rules you may follow. 1. Keep everything simple and clean Avoid complex coding in your program if you have complex program then use your time to analysis and divide into subtasks. 2. Be consistent Be consistent as much as possible whatever you do. This is especially important when writing your code against your coding rules-coding standards. 3. Have a coding standard A coding standard defines how you write your code. 1. For class names use Pascal Casing 2. For Function names use Pascal Casing 3. For Properties use Pascal Casing 4. Use camel Casing for member variables, parameters, local variables 5. To distinguish between local and member variables use this keyword and don’t use Hungarian and notations having _,m_,s_ for your local or member variables 1. Com